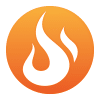
Lazy loading is a widely used technique for improving the performance of websites and web applications. Websites are becoming more visual, and images are expensive. Images and videos take significant time to download (in web terms, when 2 seconds for the entire web page is too much). They also consume significant chunks of your users’ mobile data allowance. Modern image formats have improved download sizes and quality – but lazy loading offers a significant benefit over that.
What is Lazy Loading?
It is a design pattern aimed at delaying the initialisation of resources until they are actually needed. In the context of web development, it primarily refers to the practice of deferring the loading of images and media files on web pages until they enter the user’s viewport. If an image is located at the bottom of a page and the user hasn’t scrolled to it, it won’t be loaded until the user gets there. This significantly decreases the amount of data that must be loaded when the webpage first opens, leading to improved loading times and reduced resource consumption.
How Does Lazy Loading Work?
The concept behind lazy loading for images and media is straightforward. Instead of loading all media elements as soon as the page loads, you load them only when they are needed. Here’s how you can implement lazy loading with images:
1. Using HTML:
The simplest form of lazy loading can be implemented using native HTML by taking advantage of the loading="lazy"
attribute in <img>
tags:
<img src="example.jpg" alt="Description" loading="lazy">
Code language: HTML, XML (xml)
This method is supported by most modern browsers and provides an easy-to-implement solution without any additional JavaScript.
2. Using JavaScript and Intersection Observer:
For more control over the process and for broader compatibility, the Intersection Observer API can be used. This JavaScript API enables the performance-friendly detection of when an element enters the user’s viewport. Here is a basic example:
document.addEventListener("DOMContentLoaded", function () {
var lazyImages = [].slice.call(document.querySelectorAll("img.lazy"));
if ("IntersectionObserver" in window) {
let lazyImageObserver = new IntersectionObserver(function (entries, observer) {
entries.forEach(function (entry) {
if (entry.isIntersecting) {
let lazyImage = entry.target;
lazyImage.src = lazyImage.dataset.src;
lazyImage.classList.remove("lazy");
lazyImageObserver.unobserve(lazyImage);
}
});
});
lazyImages.forEach(function (lazyImage) {
lazyImageObserver.observe(lazyImage);
});
} else {
// Fallback for older browsers
lazyImages.forEach(function (lazyImage) {
lazyImage.src = lazyImage.dataset.src;
lazyImage.classList.remove("lazy");
});
}
});
Code language: JavaScript (javascript)
In this code, images with the class “lazy” are observed by an IntersectionObserver
. When an image becomes visible in the viewport, its actual source URL (data-src
) is loaded into the src
attribute, and it is marked as no longer lazy.
Benefits of Lazy Loading Images
- Enhanced Performance: Reduces the initial load time of web pages by loading fewer data upfront.
- Improved Resource Management: Saves bandwidth for both the user and server. This is especially significant for users with limited data plans or slow network connections.
- Seamless User Experience: Delays in loading non-critical resources go unnoticed by users, improving perceived performance.
Conclusion
This is a powerful technique in the toolkit of web developers aimed at optimising content-heavy pages where images and media can overwhelm the network and processing capabilities. By implementing lazy loading, websites can ensure that they are not only faster but also more efficient in how they utilise resources, thereby delivering a better overall user experience. Whether using simple HTML or more complex JavaScript-based solutions, lazy loading should be employed wherever possible on any website.